vue cli 使用 iconfont
已知 iconfont 是非常好用的图标库,那么如何在项目里使用它呢?
创建 Iconfont 项目
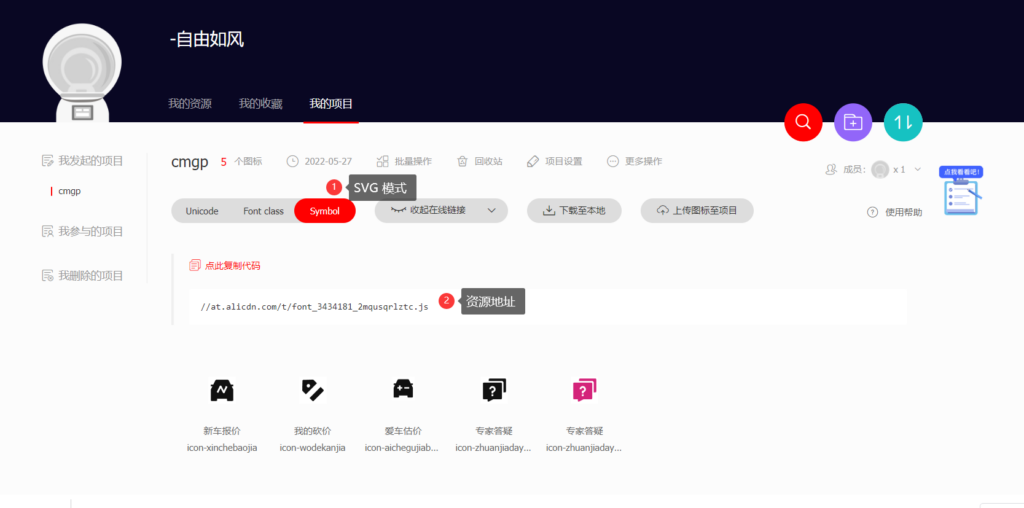
将资源引入到vue cli项目里
在 public/index.html 文件里,添加一行代码
<script src="<实际资源地址>"></script>
引入通用的css类
.icon {
width: 1em;
height: 1em;
vertical-align: -0.15em;
fill: currentColor;
overflow: hidden;
}
使用方式
<svg class="icon" aria-hidden="true">
<use :xlink:href="<实际ID>"></use>
</svg>
实际ID可以通过 iconfont 后台复制
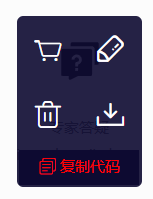
封装成组件的形式
<template>
<svg class="icon" aria-hidden="true">
<use :xlink:href="icon"></use>
</svg>
</template>
<script>
export default {
name: "IconFont",
props: {
icon: {
type: String,
required: true,
}
},
created() {
if (this.icon == undefined) {
throw new Error("使用 iconfont 组件比如传入类名");
}
}
}
</script>
<style scoped>
</style>
setup 组件
<template>
<svg class="icon-font" aria-hidden="true">
<use :xlink:href="icon"></use>
</svg>
</template>
<script lang="ts" setup>
const props = defineProps({
icon: {
type: String,
required: true,
},
});
if (props.icon === undefined) {
throw new Error("使用 IconFont 组件需要传入 icon 属性");
}
</script>
<script lang="ts">
export default {
name: "IconFont",
};
</script>
<style lang="scss" scoped>
.icon-font {
width: 1em;
height: 1em;
vertical-align: -0.15em;
fill: currentColor;
overflow: hidden;
}
</style>
使用第三方组件的形式
npm官方地址 支持的是 vue2.x
安装
# install global
npm install vue-svgicon -g
# install for project
npm install vue-svgicon --save